Getting Started
Geoflip is a powerful spatial data processing API that lets developers easily transform, reproject, and convert spatial data using a simple REST API. Let’s walk through how to use the Geoflip API.
Create an API Key
Before using Geoflip, you’ll need to generate an API key to authenticate your requests.
- Visit account.geoflip.io and sign up for a free account.
- Once signed in, navigate to the API Keys section and create an API Key to use.
- Click Create API Key, give it a name, set an expiry date and you're ready to start making requests.
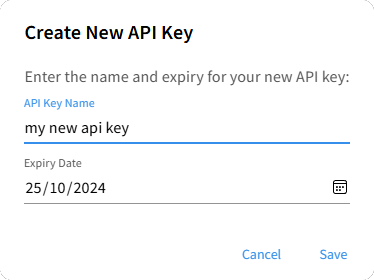
Your free account includes 30 free API calls to get you started.
Converting data and reprojecting
Geoflip makes it easy to convert and reproject spatial data in just one step, letting you switch between formats for different contexts.
For web-based GIS, GeoJSON is a popular format because it's lightweight and works well with mapping frameworks like Google Maps or Mapbox. But when you need to use desktop GIS or CAD software, formats like SHP, GPKG, and DXF are often required.
A common use case is converting GeoJSON from a web map to SHP or DXF for desktop software. Likewise, if you want to display SHP data on a web map, you'll need to convert it to GeoJSON. Geoflip makes both conversions easy.
GeoJSON to Shapefile example
Here is an example of converting GeoJSON to Shapefile, a geoflip request payload always provides some kind of input data and defines an output_format
with an output_crs
. For a GeoJSON input request we can provide the GeoJSON data in the request payload like this:
const payload = {
input_geojson: {
"type": "FeatureCollection",
"features": [
{
"id": "1",
"type": "Feature",
"properties": {},
"geometry": {
"type": "Point",
"coordinates": [115.8567, -31.9505] // Simple point geometry
}
}
]
},
output_format: "shp",
output_crs: "EPSG:4326"
};
Geoflip will respond with the Shapefile as a blob, so we need to set the responseType
of our fetch
or axios
request to blob
. Make sure to include the Geoflip API key you generated earlier in the apiKey
request header:
const response = await axios.post(
'https://api.geoflip.io/v1/transform/geojson',
JSON.stringify(payload),
{
headers: {
"Content-Type": "application/json",
"apiKey": `YOUR_API_KEY` // Replace with your actual geoflip API key
},
responseType: 'blob' // Ensure the response is treated as a file (blob)
}
);
Since the response is a blob, we’ll use window.URL.createObjectURL()
to create a link to the file for download:
if (response.status === 200) {
// Create a URL for the file download
const url = window.URL.createObjectURL(response.data);
console.log(`Shapefile download ready: ${url}`);
}
Now that we have a URL to the file, we can handle it however we like.
Below is the completed example with a download button:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>GeoJSON to Shapefile Conversion with Geoflip</title>
</head>
<body>
<h1>GeoJSON to Shapefile Conversion</h1>
<button id="convertButton">Convert GeoJSON to Shapefile</button>
<p id="statusMessage"></p>
<a id="downloadLink" style="display: none;" download="output.shp">Download Shapefile</a>
<script>
document.getElementById('convertButton').addEventListener('click', async () => {
const payload = {
input_geojson: {
"type": "FeatureCollection",
"features": [
{
"id": "1",
"type": "Feature",
"properties": {},
"geometry": {
"type": "Point",
"coordinates": [115.8567, -31.9505] // Simple point geometry
}
}
]
},
output_format: "shp",
output_crs: "EPSG:4326"
};
document.getElementById('statusMessage').textContent = 'Converting...';
try {
const response = await axios.post(
'https://api.geoflip.io/v1/transform/geojson',
JSON.stringify(payload),
{
headers: {
"Content-Type": "application/json",
"apiKey": `YOUR_API_KEY`, // Replace with your actual Geoflip API key
},
responseType: 'blob' // Ensure the response is treated as a file (blob)
}
);
if (response.status === 200) {
// Create a URL for the file download
const url = window.URL.createObjectURL(response.data);
// Display the download link
const downloadLink = document.getElementById('downloadLink');
downloadLink.href = url;
downloadLink.style.display = 'block';
downloadLink.textContent = 'Download Shapefile';
document.getElementById('statusMessage').textContent = 'Conversion successful! Your download is ready.';
}
} catch (error) {
console.error('Error converting GeoJSON to Shapefile:', error);
document.getElementById('statusMessage').textContent = 'Error during conversion.';
}
});
</script>
<!-- Include Axios for making HTTP requests -->
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
</body>
</html>
Shapefile to GeoJSON Example
Geoflip can take spatial files like Shapefiles and convert them into GeoJSON or other formats. To send a file to Geoflip, we use multipart form data. Here's an example of how to upload a Shapefile and convert it to GeoJSON using the API:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Shapefile to GeoJSON Conversion with Geoflip</title>
</head>
<body>
<h1>Shapefile to GeoJSON Conversion</h1>
<input type="file" id="fileInput" accept=".shp" />
<button id="uploadButton">Upload and Convert Shapefile</button>
<p id="statusMessage"></p>
<pre id="geojsonOutput"></pre>
<script>
document.getElementById('uploadButton').addEventListener('click', async () => {
const selectedFile = document.getElementById('fileInput').files[0];
if (!selectedFile) {
document.getElementById('statusMessage').textContent = "Please select a Shapefile.";
return;
}
document.getElementById('statusMessage').textContent = "Converting...";
try {
const formData = new FormData();
formData.append('file', selectedFile);
const config = {
output_format: "geojson", // We're converting to GeoJSON
output_crs: "EPSG:4326" // Specify the output CRS
};
formData.append('config', JSON.stringify(config));
const response = await axios.post(
'https://api.geoflip.io/v1/transform/shp', // Endpoint for shapefile conversion
formData,
{
headers: {
'Content-Type': 'multipart/form-data',
"apiKey": `YOUR_API_KEY` // Replace with your actual API key
}
}
);
if (response.status === 200) {
const geojsonData = response.data;
console.log("Shapefile successfully converted to GeoJSON:", geojsonData);
// Display the GeoJSON data in the <pre> element
document.getElementById('geojsonOutput').textContent = JSON.stringify(geojsonData, null, 2);
document.getElementById('statusMessage').textContent = "Conversion successful!";
}
} catch (error) {
console.error('Error uploading and converting Shapefile to GeoJSON:', error);
document.getElementById('statusMessage').textContent = "Error during conversion.";
}
});
</script>
<!-- Include Axios for making HTTP requests -->
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
</body>
</html>
In this case, the Shapefile should be provided as a ZIP file containing the SHP, SHX, PRJ, and DBF files. The output_format
and output_crs
are defined in the config
part of the form request, while the ZIP file is included in the file
part of the request.
Since we are requesting GeoJSON as the output format, Geoflip will respond with a simple JSON response containing the converted data.
Applying Transformations
If you want to apply spatial transformations to your input data before converting it to your specified output format, you can define them in the config
or the request payload
using the transformations
key:
const payload = {
input_geojson: {
"type": "FeatureCollection",
"features": [
{
"id": "1",
"type": "Feature",
"properties": {},
"geometry": {
"type": "Point",
"coordinates": [115.8567, -31.9505] // Simple point geometry
}
}
]
},
output_format: "shp",
output_crs: "EPSG:4326",
transformations: [
{
"type":"buffer",
"distance": 100,
"units": "meters"
},
{
"type":"union"
}
]
};
In the example payload above, the data is supplied as GeoJSON, and we’ve requested the output in Shapefile format. Before the data is returned, we also apply two transformations: a 100-meter buffer around the point and a union operation using the buffer
and union
transformations.
To learn more about Geoflip transformations, refer to the Transformations page.
Handling large files with async
To handle larger, longer-running jobs, Geoflip offers async requests by adding the ?async=true
URL parameter to any API request. By doing this, Geoflip will immediately respond with a task_id instead of processing the request synchronously:
{
"message": "Geoflip GPKG task as been created",
"state": "TASK CREATED",
"task_id": "cec5b73c-b70a-4c23-88f6-7c50e212ad5d"
}
Using this task_id
, you can make subsequent requests to Geoflip to check the status of the geoprocessing task via the async result endpoint:
https://api.geoflip.io/v1/transform/result/{{taskid}}
If the task is still in progress, Geoflip will return the current state of the job. Once it is completed, Geoflip will provide a download URL:
{
"message": "Task completed successfully",
"output_url": "https://api.geoflip.io/v1/transform/output/o_cec5b73c-b70a-4c23-88f6-7c50e212ad5d",
"state": "SUCCESS"
}
Please note, this URL will only work once. As soon as the URL is accessed and the data is successfully retrieved, the source data will be deleted and the URL will no longer be accessible.
Free account limitations
With Geoflip's free account, users enjoy the full functionality of the API, but there are a couple of important limitations:
Request Throttling: Free accounts are limited to 1 request per second. This ensures fair usage across the platform.
Free API Calls: Upon signup, you receive 30 free API calls to get started. After these free calls are used, you can switch to the pay-as-you-go pro account to continue using the service.
Other than these, there are no restrictions on the features you can access with the free account so feel free to test out the various transformations and data conversions.